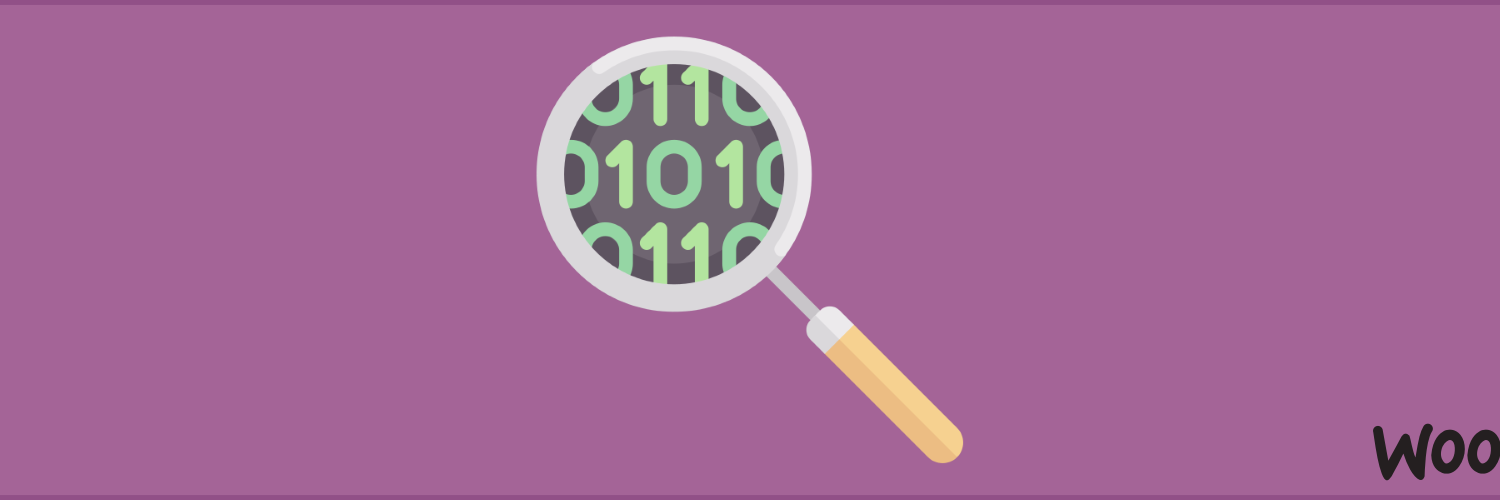
How to Add MPN to WooCommerce
In the current article, we will review how to add a unique number issued by manufacturers to identify individual products – Manufacturer Part Number (MPN) – to your products in WooCommerce. We will describe how to add and save it for your products and variations in the backend; how to display MPNs for products and variations in the frontend; and how to add MPNs to your products schema.
Adding MPN to WooCommerce
MPN field
First of all, we need a field in our backend for the MPN. The snippet below will add the MPN field right after the standard SKU field, and it will save the field’s value when we update the product. You can change the position of our MPN field by replacing the woocommerce_product_options_sku
with some other hook, for example, if you’ll use woocommerce_product_options_pricing
, then the field will be added right after the product pricing fields.
add_action( 'woocommerce_product_options_sku', 'wpfactory_add_mpn' ); add_action( 'save_post_product', 'wpfactory_save_mpn', 10, 2 ); if ( ! function_exists( 'wpfactory_add_mpn' ) ) { /** * Adds MPN field to product admin edit page. */ function wpfactory_add_mpn() { woocommerce_wp_text_input( array( 'id' => 'mpn', 'value' => get_post_meta( get_the_ID(), 'mpn', true ), 'label' => esc_html__( 'MPN', 'text-domain' ), ) ); } } if ( ! function_exists( 'wpfactory_save_mpn' ) ) { /** * Saves product MPN field. */ function wpfactory_save_mpn( $post_id, $__post ) { if ( isset( $_POST['mpn'] ) && empty( $_REQUEST['woocommerce_quick_edit'] ) ) { update_post_meta( $post_id, 'mpn', wc_clean( $_POST['mpn'] ) ); } } }
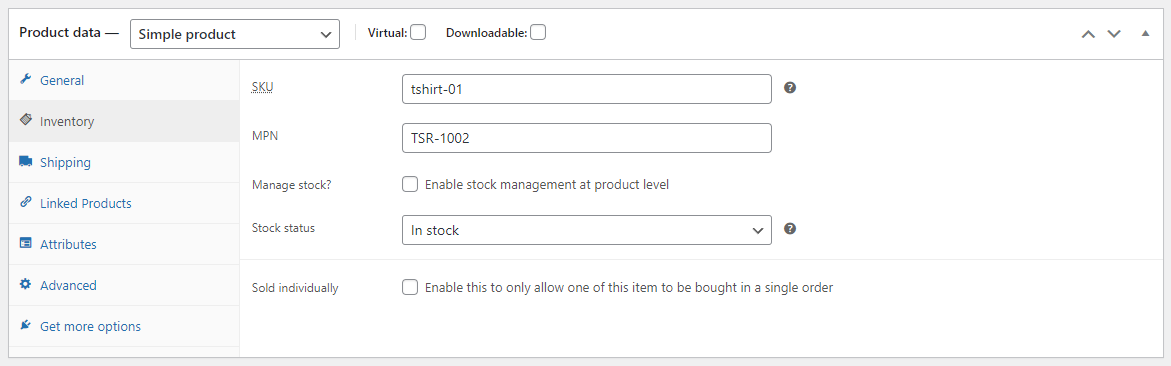
Variations
You probably noticed that MPN is not added for variable product variations. To add it to each variation, we need to add an extra snippet. Like the first snippet, it will add the field and save it on product updates. You can change the field’s position by replacing the woocommerce_variation_options_pricing
hook. For example, if you use the woocommerce_variation_options_dimensions
hook, the field will be added right after the variation’s dimensions fields.
add_action( 'woocommerce_variation_options_pricing', 'wpfactory_add_mpn_variation', 10, 3 ); add_action( 'woocommerce_save_product_variation', 'wpfactory_save_mpn_variation', 10, 2 ); if ( ! function_exists( 'wpfactory_add_mpn_variation' ) ) { /** * Adds MPN field to variations. */ function wpfactory_add_mpn_variation( $loop, $variation_data, $variation ) { woocommerce_wp_text_input( array( 'id' => "variable_mpn_{$loop}", 'name' => "variable_mpn[{$loop}]", 'value' => ( isset( $variation_data['mpn'][0] ) ? $variation_data['mpn'][0] : '' ), 'label' => esc_html__( 'MPN', 'text-domain' ), 'wrapper_class' => 'form-row form-row-full', ) ); } } if ( ! function_exists( 'wpfactory_save_mpn_variation' ) ) { /** * Saves variation MPN field. */ function wpfactory_save_mpn_variation( $variation_id, $i ) { if ( isset( $_POST['variable_mpn'][ $i ] ) ) { update_post_meta( $variation_id, 'mpn', wc_clean( $_POST['variable_mpn'][ $i ] ) ); } } }
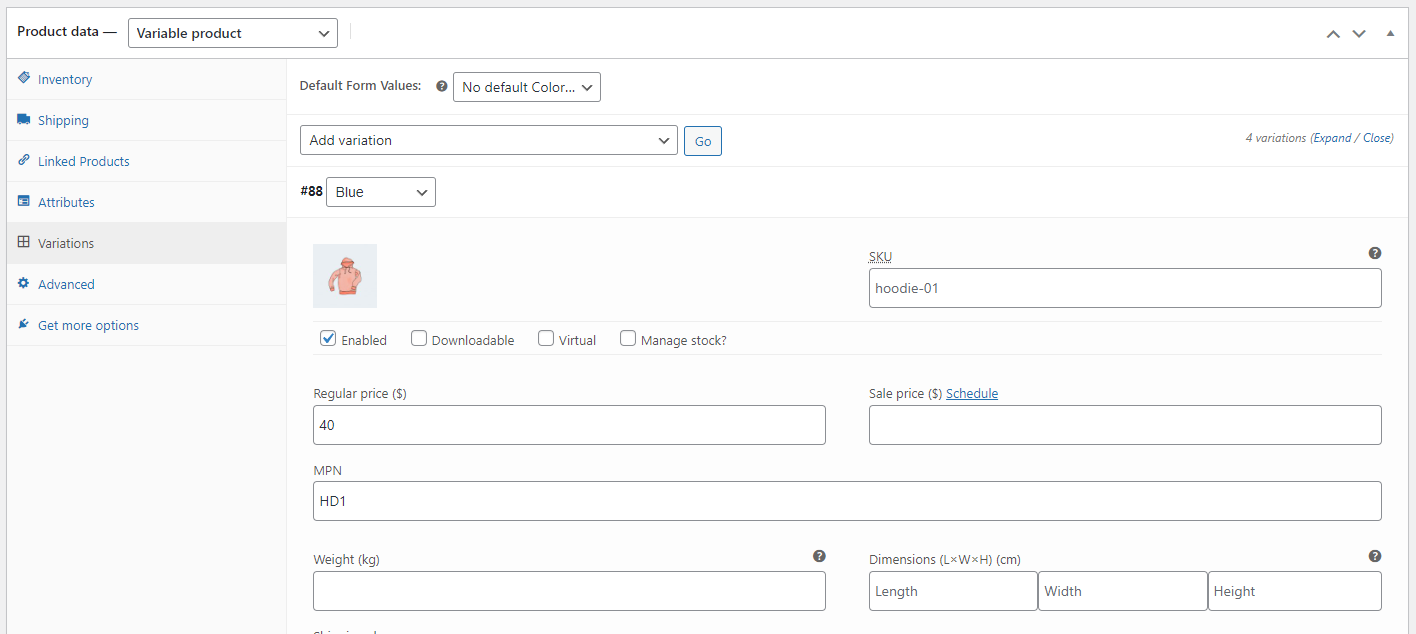
Display
Currently, the MPN field is only visible in the backend. If you want to display it to your customers on the frontend, use the snippet below. It will add the MPN to the product meta, right above the SKU. If you want to display it at the end of the product meta, replace the woocommerce_product_meta_start
hook with woocommerce_product_meta_end
.
add_action( 'woocommerce_product_meta_start', 'wpfactory_display_mpn' ); if ( ! function_exists( 'wpfactory_display_mpn' ) ) { /** * Display MPN. */ function wpfactory_display_mpn() { if ( '' !== ( $mpn = get_post_meta( get_the_ID(), 'mpn', true ) ) ) { printf( '<span class="mpn_wrapper">%s: <span class="mpn">%s</span></span>', esc_html__( 'MPN', 'text-domain' ), $mpn ); } } }
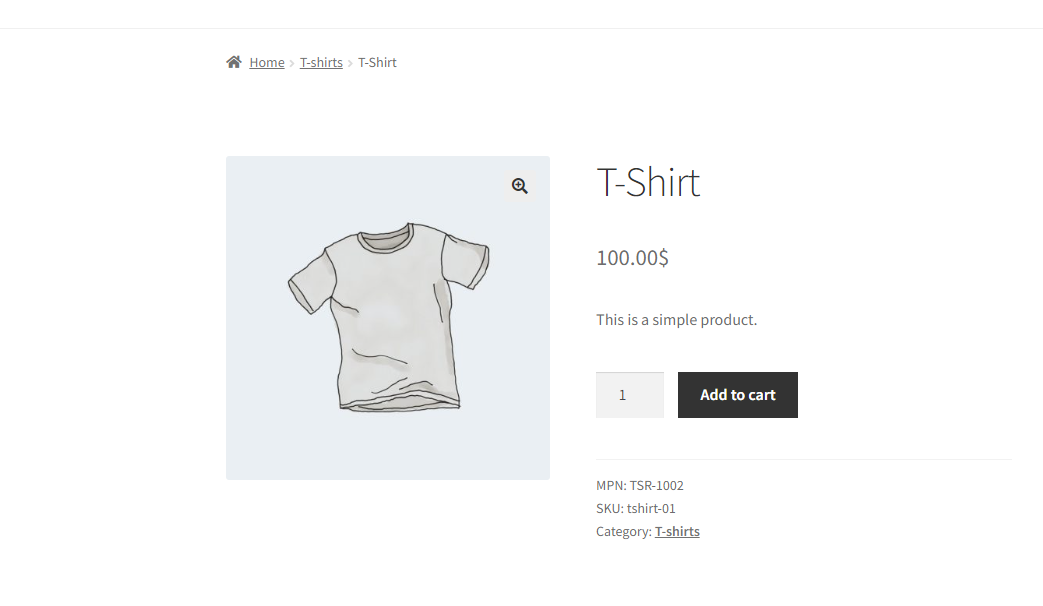
Display for variations
Like the backend, we’ll need a separate snippet to handle variations on the frontend. The easiest way is to add the MPN to each variation’s description – this way, it will be automatically updated when a user selects a different variation.
add_filter( 'woocommerce_available_variation', 'wpfactory_display_mpn_variation', 10, 3 ); if ( ! function_exists( 'wpfactory_display_mpn_variation' ) ) { /** * Display MPN in variation description. */ function wpfactory_display_mpn_variation( $args, $product, $variation ) { if ( '' !== ( $mpn = $variation->get_meta( 'mpn' ) ) ) { $args['variation_description'] .= sprintf( '<span class="mpn_wrapper">%s: <span class="mpn">%s</span></span>', esc_html__( 'MPN', 'text-domain' ), $mpn ); } return $args; } }
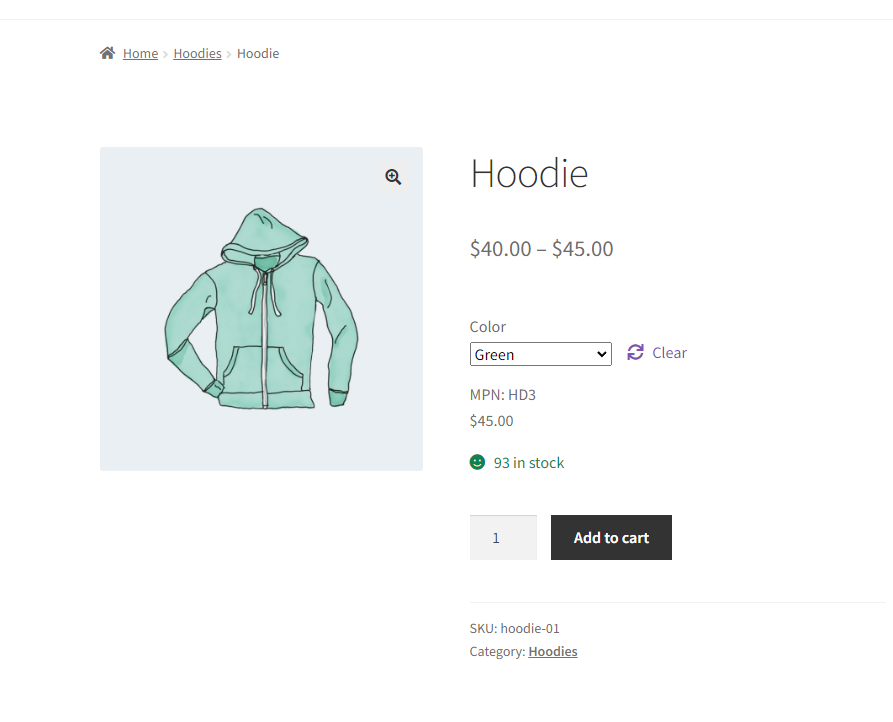
Product structured data
Finally, you may want to add the MPN to the product schema.
add_filter( 'woocommerce_structured_data_product', 'wpfactory_add_mpn_structured_data_product', 10, 2 ); if ( ! function_exists( 'wpfactory_add_mpn_structured_data_product' ) ) { /** * Adds MPN field to product structured data. */ function wpfactory_add_mpn_structured_data_product( $markup, $product ) { if ( '' !== ( $mpn = get_post_meta( $product->get_id(), 'mpn', true ) ) ) { $markup['mpn'] = $mpn; } return $markup; } }
Hi,
I used your code and it works great. But how can i make the MPN work on the search results?
Thanks
Hi, Goncalo,
Sorry for not getting back to you sooner.
Please try this:
Hi and thanks a lot for the code. It works PERFECT!!!
I have a question. Is it possible to manage the position where MPN field is shown on admin-edit product page?
For example, now in Inventory tab 1st box is SKU, 2nd box is something else and box for MPN is 5th. Can i mov MPN to 1st box, SKU to 2nd?
Hi,
Happy to help ๐
Yes, it’s possible, but I think the only way is JavaScript.
Please try adding this to your code:
I hope this helps.
Hi Tom,
I am trying to use for a variable product by adding the variable code to function.php and this works.
I would like the this mpn to appear in the data so that I can pick it up in Product Feed Pro to use with Google shopping. I copied the last bit of code in function.php at the very end but I don’t seem to pick it up in Product Feed
Any help please?
Hi,
It would be better if you ask the product feed plugin developers directly, but I think you need to set the MPN for at least one of your products, and then you should be able to select the “Mpn (Custom attribute)” when adding a custom field in the feed’s mapping settings.
Hi Tom,
It is working now as I think it was a cached version of display I was looking at, clearing it the “Mpn (Custom attribute)โ is popping up correctly.
I am using your snippet code of MPN for product variation, and I wanted it to come up at the end and I used your suggestion of placing it end of product meta. However, when I select a particular variation of the product the MPN seems to print directly below the variation selection box. The rest of the product meta (including SKU, GTIN Category, Tags etc) is below the “Add to Basket” selection button.
Wish I could attach a screen shot but don’t know how to here.
Any solution you could suggest for bundling up all the product meta together?
Hi,
> … the โMpn (Custom attribute)โ is popping up correctly
Happy to hear it’s solved ๐
> I am using your snippet code of MPN for product variation…
Unfortunately, the “product meta” actions do not affect variations. As variations can be switched dynamically on the front end, we need JavaScript to update MPN. The easiest way is to use what’s already implemented in WooCommerce – variation description is updated automatically when a different variation is selected – so we add our MPN to the description.
Very useful guide!
what is the meta field name that can be used for bulk upload ???
Hi Avraham,
Happy to help ๐
We used the
mpn
meta key in our snippets.I hope this helps. Please let me know if you have any questions.
Thank you, now if you could just a cost field for when the cost price is also a sale price in the cost plugin.
Hi,
I’m not sure if I got it correctly – could you please elaborate?
Where do these snippets go?
Hi Frances,
You can add it to your (child) theme’s functions.php file, or use a plugin, e.g., Code Snippets.
P.S. You can also check our How to Add Custom PHP Code in WordPress article.